Python in Terminal ↩
Python can be used directly in the console as a command-line interface. On macOS, this is done using the Terminal – you can find it in your Applications / Utilities folder.
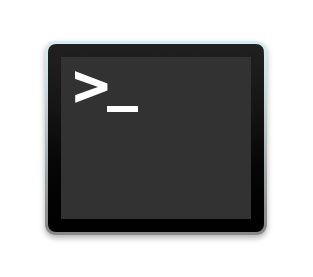
After launching Terminal, you will see something like this:
Last login: Wed Jul 23 05:01:17 on ttys000
username:~
This is your default command-line prompt, with your own username.
There are two ways to use Python in the console:
- interactive mode
- running existing scripts
Interactive mode
To enter interactive mode in Terminal, simply type python
in the command-line:
username:~ python
You will probably see something like this:
Python 2.7.5 (default, Aug 25 2013, 00:04:04)
[GCC 4.2.1 Compatible Apple LLVM 5.0 (clang-500.0.68)] on darwin
Type "help", "copyright", "credits" or "license" for more information.
This command gives us some information about the current Python installation, and returns an interactive Python prompt.
The default Python in macOS is still Python 2.7.X. See Installing Python 3 on your system if you wish to use Python 3 in Terminal.
Once in the Python prompt, we can start typing code, and it will get executed.
Let’s start with a simple hello world:
>>> "hello world"
'hello world'
In interactive mode, it is not necessary to use the
print()
function – every expression is executed and returns its result.
Throughout this documentation, code examples with lines starting in
>>>
are intended as interactive sessions in Terminal. All other examples are scripts.
The same is valid for mathematical expressions (or for any Python expression):
>>> 1 + 1
2
>>> 'x' in 'abcdef'
False
It’s also possible to write multi-line code in interactive mode. Notice how the prompt changes to indicate that the interpreter is waiting for input:
>>> for i in range(4):
... i
...
0
1
2
3
User input
Interactive mode allows us to prompt the user for input while we are running a programming session. This can be done using the built-in function input()
, which returns the string that was entered by the user.
>>> answer = input('What… is your quest?' )
Running existing scripts
The interactive mode is useful for writing quick tests and short scripts, but it is not really suitable for working with larger pieces of code. In that case, it makes more sense to write scripts as separate .py
files, and use Terminal only to run them.
As an example, let’s suppose we have a Python script which prints hello world
as output:
print('hello world')
This script is saved in the desktop as hello.py
.
To execute this file in Terminal we use the command python
followed by the path to the script file:
username:~ python /Users/username/Desktop/hello.py
Instead of typing out the full path, you can also drag the file from Finder to the Terminal prompt to get its path.
The output will be, as expected:
hello world