Draw info text in the Glyph View ↩
This example shows how to draw informative text into the Glyph Editor canvas using merz
. Two labels with date and time since the last edit in the glyph are updated using subscriber
.
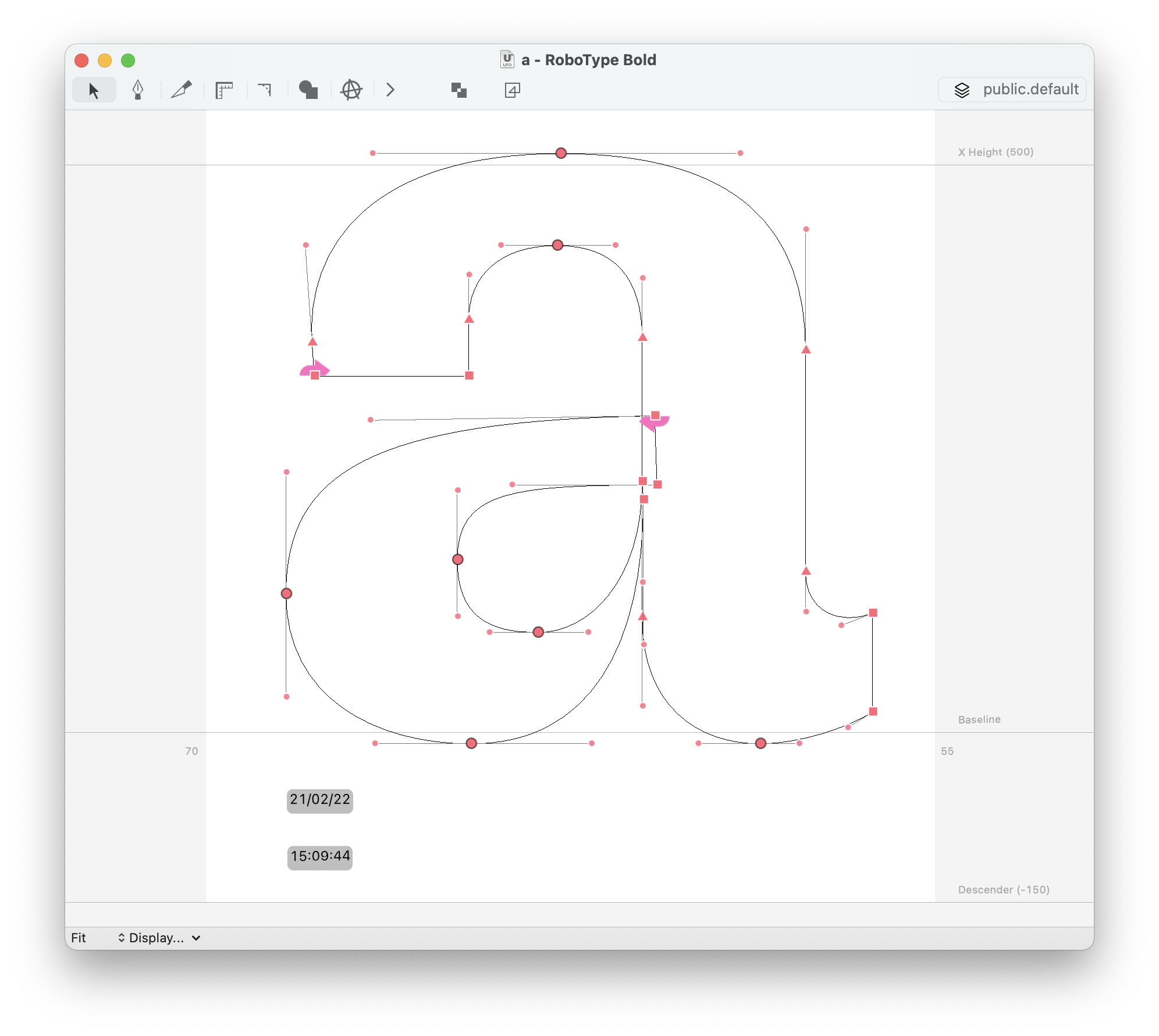
from datetime import datetime
from mojo.subscriber import Subscriber, registerGlyphEditorSubscriber
class DrawTextAtPointExample(Subscriber):
debug = True
def build(self):
glyphEditor = self.getGlyphEditor()
self.container = glyphEditor.extensionContainer(
identifier="com.roboFont.DrawTextAtPointExample.foreground",
location="foreground",
clear=True
)
self.lastEditDateLayer = self.container.appendTextLineSublayer(
position=(100, -50),
pointSize=12,
backgroundColor=(0, 0, 0, 0.25),
fillColor=(0, 0, 0, 1),
horizontalAlignment="center",
cornerRadius=5,
padding=(2, 1)
)
self.lastEditTimeLayer = self.container.appendTextLineSublayer(
position=(100, -100),
pointSize=12,
backgroundColor=(0, 0, 0, 0.25),
fillColor=(0, 0, 0, 1),
horizontalAlignment="center",
cornerRadius=5,
padding=(2, 1)
)
self.updateTexts()
def destroy(self):
self.container.clearSublayers()
def glyphDidChange(self, info):
self.updateTexts()
def glyphEditorDidSetGlyph(self, info):
self.updateTexts()
def updateTexts(self):
now = datetime.now()
self.lastEditDateLayer.setText(f"{now:%d/%m/%y}")
self.lastEditTimeLayer.setText(f"{now:%H:%M:%S}")
if __name__ == '__main__':
registerGlyphEditorSubscriber(DrawTextAtPointExample)