Custom Inspector section ↩
This example shows how to add a custom section to the Inspector panel.
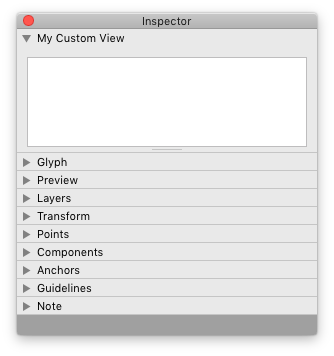
from vanilla import TextEditor
from mojo.subscriber import Subscriber, registerRoboFontSubscriber
class CustomInspectorToolbarExample(Subscriber):
debug = True
def build(self):
self.editor = TextEditor((10, 10, -10, -0))
def roboFontWantsInspectorViews(self, info):
# create an inspector item
item = dict(label="My Custom View", view=self.editor)
# insert or append the item to the list of inspector panes
info["viewDescriptions"].insert(0, item)
def glyphEditorWantsToolbarItems(self, info):
# create a toolbar item
item = dict(itemIdentifier="customGlyphToolbar",
label="Do It",
callback=self.itemCallback,
imageNamed="toolbarRun")
# insert or append the item to the list of glyph window toolbar items
info["itemDescriptions"].insert(-2, item)
def fontDocumentWantsToolbarItems(self, info):
# create a toolbar item
item = dict(itemIdentifier="customFontToolbar",
label="Do It",
callback=self.itemCallback,
imageNamed="toolbarRun")
# insert or append the item to the list of font window toolbar items
info["itemDescriptions"].insert(2, item)
def itemCallback(self, sender):
print("callback!")
if __name__ == '__main__':
registerRoboFontSubscriber(CustomInspectorToolbarExample)